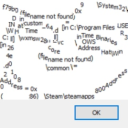
A Hat in Time Script Repository
(v0.5)
Code viewer
SS_PlatformDisappear_Extended
#CustomActor
Similar to the original Hat_PlatformDisappear, now with Sounds and Particles.
Has 2 sounds, one plays when its touched the other plays when its shrinking, same stuff with the 2 particles.
Requires SS_Platform_Base for it to work, without this file it will cause problems!
/** * * Copyright 2012-2015 Gears for Breakfast ApS. All Rights Reserved. */ class SS_Platform_Base extends Actor abstract; var(Particles) ParticleSystem ActivationParticle; var(Particles) ParticleSystem DisappearParticle; var(Sounds) SoundCue ActivationSound; var(Sounds) SoundCue DisappearSound; defaultproperties { TickOptimize = TickOptimize_View; bNoEncroachCheck = true; RemoteRole=ROLE_SimulatedProxy ActivationParticle = None; DisappearParticle = None; ActivationSound = None; DisappearSound = None; } simulated event Attach( Actor Other ) { } // True to override jump functionality simulated function bool JumpBoost(Actor other, out Vector v) { return false; } simulated function bool CallInteractionEvent(Actor other) { local int idx; local Hat_SeqEvent_OnInteraction hEvent; local bool s; s = false; // search for any events for (idx = 0; idx < GeneratedEvents.Length; idx++) { hEvent = Hat_SeqEvent_OnInteraction(GeneratedEvents[idx]); if (hEvent != None) { // notify that we have a interaction event if (hEvent.HandleInteraction(self, other)) { s = true; } } } return s; } function Vector GetCenter() { local Vector v; v = Location + vect(0,0,1)*40; return v; }
/** * * Copyright 2012-2015 Gears for Breakfast ApS. All Rights Reserved. */ class SS_PlatformDisappear_Extended extends SS_Platform_Base placeable; var() StaticMeshComponent Mesh; var() const editconst LightEnvironmentComponent LightEnvironment; var() float DisappearTime; var() float DisappearDelay; var() float DisappearDuration; var float Scale; var bool Activated; var bool IsScalingDown; defaultproperties { Begin Object Class=StaticMeshComponent Name=Model0 bUsePrecomputedShadows=TRUE LightingChannels = (Static=True,Dynamic=True) End Object Mesh=Model0 CollisionComponent=Model0 Components.Add(Model0) bEdShouldSnap=true; bWorldGeometry=false bBumpEvenIfWorldGeometry=true bCollideActors=true bBlockActors=true DisappearTime = 3; DisappearDelay = 1; DisappearDuration = 5; Scale = 1.0; TickOptimize = TickOptimize_None RemoteRole=ROLE_SimulatedProxy ActivationParticle = None; DisappearParticle = None; ActivationSound = None; DisappearSound = None; } simulated event PostBeginPlay() { Super.PostBeginPlay(); SetTickIsDisabled(true); } simulated event Attach( Actor Other ) { Super.Attach(Other); if (Activated) return; if (other.IsA('Hat_PawnGhost')) return; Activated = true; IsScalingDown = false; if (ActivationSound != None) PlaySound(ActivationSound); if (ActivationParticle != None) Worldinfo.MyEmitterPool.SpawnEmitter(ActivationParticle, GetCenter()); Scale = 1.0; SetTickIsDisabled(false); } simulated event Tick(float d) { Super.Tick(d); if (!Activated) { Scale += (1.0 - Scale)*FMin(d*5,1.0); Mesh.SetScale(Scale); return; } if (DisappearDelay > 0) { DisappearDelay -= d; if (DisappearDelay > 0) { Scale = 1.0 + Sin((default.DisappearDelay - DisappearDelay)*50)*0.05; } } if (DisappearDelay <= 0) { if (Scale > 0) { Scale -= d * (1.0/DisappearTime); if (Scale <= 0) { SetHidden(true); } } if (Scale <= 0){ if (IsScalingDown == false){ if (DisappearSound != None) PlaySound(DisappearSound); if (DisappearParticle != None) Worldinfo.MyEmitterPool.SpawnEmitter(DisappearParticle, GetCenter()); IsScalingDown = true; } DisappearDuration -= d; if (DisappearDuration <= 0){ Restart(); } } } if (Scale > 0.0) Mesh.SetScale(Scale); } function Restart() { SetHidden(false); Activated = false; DisappearDuration = default.DisappearDuration; DisappearDelay = default.DisappearDelay; Scale = 1.0; Mesh.SetScale(Scale); SetTickIsDisabled(true); }