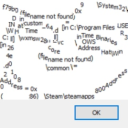
A Hat in Time Script Repository
(v0.5)
Code viewer
Find Closest Actor (Kismet)
#Kismet
Modified @ 2024-01-11 18:20:37
Finds the closest actor to a reference actor, from a list of actors.
As stated, this will search through a list of actors, and find the closest actor to the reference actor you choose. Very handy!
The variables:
Target = The reference actor. (Object)
Points = The list of actors (Object/List)
Result = The resulting closest actor, outputted to a connected object variable.
/** * * Copyright 2021 Gears for Breakfast ApS. All Rights Reserved. */ class Ink_SeqAct_FindClosestActor extends SequenceAction; var() Actor Target; var() Array<Actor> Points; var() bool IgnoreZ; var Actor ActorResult; event Activated() { local Actor CurrPoint, BestTarget; local float CurrentDist, BestDist; if (Target != None && Target.IsA('Controller') && Controller(Target).Pawn != None) Target = Controller(Target).Pawn; if (Target != None && Points.Length > 0) { foreach Points(CurrPoint) { if (CurrPoint == None) continue; if (Controller(CurrPoint) != None && Controller(CurrPoint).Pawn != None) CurrPoint = Controller(CurrPoint).Pawn; CurrentDist = VSizeSq((CurrPoint.Location - Target.Location)*(IgnoreZ ? Vect(1,1,0) : Vect(1,1,1))); if (BestTarget != None && CurrentDist >= BestDist) continue; BestTarget = CurrPoint; BestDist = CurrentDist; } } else BestTarget = None; ActorResult = BestTarget; ActivateOutputLink(0); } static event int GetObjClassVersion() { return Super.GetObjClassVersion() + 1; } defaultproperties { ObjName="Find Closest Actor (Template)" ObjCategory="Ink Kismet" bCallHandler=false; bAutoActivateOutputLinks=false; VariableLinks.Empty; VariableLinks(0)=(ExpectedType=class'SeqVar_Object', LinkDesc="Target", PropertyName=Target); VariableLinks(1)=(ExpectedType=class'SeqVar_Object', LinkDesc="Points", PropertyName=Points); VariableLinks(2)=(ExpectedType=class'SeqVar_Object', LinkDesc="Result", PropertyName=ActorResult, bWriteable=TRUE); }