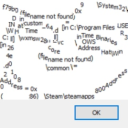
A Hat in Time Script Repository
(v0.5)
Code viewer
AnimNotify - PlayCameraAnim
Use this animation notify to apply an animation to the player camera! Seamless cutscenes!
This custom animation notify allows you to play a CameraAnim, either in the current player camera, or by creating a brand new one. Some examples of what this could be used for include taunts with custom camera movement, cutscenes, camera shakes, etc...
Some features:
- Blend in and out
- Camera blending like in kismet
- Cinematic mode options, including new ones like hiding the cinematic black bars
- Forcing camera positions and rotations
- Anim rate and scale modifiers
class Hat_AnimNotify_PlayCameraAnim extends AnimNotify_Scripted; /* This custom animation notify allows you to play CameraAnims directly from animations in an AnimSet, without the need for a separate script or kismet setup. The option CreateNewCamera should be used if this notify is being played in a non-player mesh, in case of use in a cutscene, for example. The variable tooltips go into further detail as for this notifies options. UltraBoo 2024 */ var array<CameraAnimInst> animInst; var DynamicCameraActor tempCamera; struct CinematicModeParams { var() bool bHidePlayer<ToolTip=Should we hide the player? Can not be used if owner is a player.>; var() bool bAffectsHUD<ToolTip=Should the HUD be hid?>; var() bool bAffectsMovement<ToolTip=If true, the player won't be able to move.>; var() bool bAffectsTurning<ToolTip=If true, the player won't be able to rotate the camera>; var() bool bAffectsButtons<ToolTip=If true, the player's inputs won't be registered, for the most part.>; var() bool bNewCamRot<ToolTip=If the camera should be set to a new rotation. Only applicable if using the player camera.>; var() bool bHideCinematicBars<ToolTip=Should the cinematic mode black bars be hidden?>; }; var() CameraAnim CameraAnimation<ToolTip=The CameraAnim to be used.>; var() float AnimRate<ToolTip=How fast the animation should play.>; var() float AnimMoveScale<ToolTip=Scales the movement of the animation by this factor.>; var() Vector2D BlendInOutTime<ToolTip=The Blend In and Blend Out time values, in seconds.>; var() bool UseCinematicMode<ToolTip=Activates cinematic mode. Needed if player lock or hiding player is needed.>; var() CinematicModeParams CinematicModeSettings<ToolTip=Settings for the cinematic mode>; var() bool CreateNewCamera<ToolTip=Creates a new camera instead of using the player's camera. Must be on if CameraAnim is to be played outside the player model.>; var(NewCamera) Rotator NewCameraRotation<ToolTip=Forces player camera to start at certain rotation. Player Camera Lock or New Camera is required to be true.>; var(NewCamera) Vector NewCameraPosition<ToolTip=Places camera at certain offset. New Camera is required to be true.>; var(NewCamera) ViewTargetTransitionParams NewCamSettings<ToolTip=Settings for the transition between the player camera and the new camera.>; event Notify(Actor Owner, AnimNodeSequence AnimSeqInstigator) { local Hat_PlayerController plyControl; local WorldInfo WI; local bool canHideCinematic; local int i; if(CameraAnimation == none) return; if(Hat_Player(Owner) != none && CinematicModeSettings.bHidePlayer) canHideCinematic = true; else canHideCinematic = false; i = 0; if(CreateNewCamera) { tempCamera = Owner.Spawn(class'DynamicCameraActor',,, Owner.Location + QuatRotateVector(QuatFromRotator(Owner.Rotation),NewCameraPosition), Owner.Rotation + NewCameraRotation,,true); WI = class'WorldInfo'.static.GetWorldInfo(); foreach WI.AllControllers(class'Hat_PlayerController', plyControl) { plyControl.PlayerCamera.SetViewTarget(tempCamera, NewCamSettings); animInst[i] = plyControl.PlayerCamera.PlayCameraAnim(CameraAnimation,AnimRate,AnimMoveScale,BlendInOutTime.X, BlendInOutTime.Y, false, false); if(UseCinematicMode) { if(CinematicModeSettings.bHideCinematicBars) plyControl.HideCinematicBars = true; plyControl.SetCinematicMode(true,canHideCinematic,CinematicModeSettings.bAffectsHUD,CinematicModeSettings.bAffectsMovement,CinematicModeSettings.bAffectsTurning,CinematicModeSettings.bAffectsButtons); if (class'Hat_HUDMenu_SwapHat'.static.IsOpen(plyControl)) Hat_Player(plyControl.Pawn).BadgeSwitchDeactivate(); } i++; } } else { if (Hat_Player(Owner) == None) return; plyControl = Hat_PlayerController(Hat_Player(Owner).Controller); animInst[0] = plyControl.PlayerCamera.PlayCameraAnim(CameraAnimation,AnimRate,AnimMoveScale,BlendInOutTime.X, BlendInOutTime.Y, false, false); if(UseCinematicMode) { if(CinematicModeSettings.bNewCamRot) plyControl.SetRotation(Owner.Rotation + NewCameraRotation); if(CinematicModeSettings.bHideCinematicBars) plyControl.HideCinematicBars = true; plyControl.SetCinematicMode(true,false,CinematicModeSettings.bAffectsHUD,CinematicModeSettings.bAffectsMovement,CinematicModeSettings.bAffectsTurning,CinematicModeSettings.bAffectsButtons); if (class'Hat_HUDMenu_SwapHat'.static.IsOpen(plyControl)) Hat_Player(plyControl.Pawn).BadgeSwitchDeactivate(); } } } event NotifyEnd( Actor Owner, AnimNodeSequence AnimSeqInstigator ) { local Hat_PlayerController plyControl; local WorldInfo WI; local bool canHideCinematic; local int i; if(animInst.Length <= 0) return; if(Hat_Player(Owner) != none && CinematicModeSettings.bHidePlayer) canHideCinematic = true; else canHideCinematic = false; i = 0; if(CreateNewCamera) { WI = class'WorldInfo'.static.GetWorldInfo(); foreach WI.AllControllers(class'Hat_PlayerController', plyControl) { plyControl.PlayerCamera.SetViewTarget(plyControl.ViewTarget, NewCamSettings); plyControl.PlayerCamera.StopCameraAnim(animInst[i], true); if(UseCinematicMode) { plyControl.SetCinematicMode(false,canHideCinematic,CinematicModeSettings.bAffectsHUD,CinematicModeSettings.bAffectsMovement,CinematicModeSettings.bAffectsTurning,CinematicModeSettings.bAffectsButtons); if(CinematicModeSettings.bHideCinematicBars) plyControl.HideCinematicBars = false; } i++; } tempCamera.Destroy(); tempCamera = none; } else { if (Hat_Player(Owner) == None) return; plyControl = Hat_PlayerController(Hat_Player(Owner).Controller); plyControl.PlayerCamera.StopCameraAnim(animInst[0], true); if(UseCinematicMode) { plyControl.SetCinematicMode(false,false,CinematicModeSettings.bAffectsHUD,CinematicModeSettings.bAffectsMovement,CinematicModeSettings.bAffectsTurning,CinematicModeSettings.bAffectsButtons); if(CinematicModeSettings.bHideCinematicBars) plyControl.HideCinematicBars = false; } } AnimInst.Length = 0; } defaultproperties { NotifyColor=(R=100,G=255,B=170,A=255); AnimRate = 1; AnimMoveScale = 1; BlendInOutTime = (X = 0, Y = 0); UseCinematicMode = true; NewCameraRotation = (Pitch = 0, Yaw = 0, Roll = 0); NewCameraPosition = (X = 0, Y = 0, Z = 0); CreateNewCamera = false; }