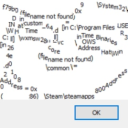
A Hat in Time Script Repository
(v0.5)
Code viewer
Hat_AnimNotify_PawnPlayVoice
Modified @ 2025-04-09 12:10:10
AnimNotify that calls PlayVoice function if Owner is Hat_Pawn.
class Hat_AnimNotify_PawnPlayVoice extends AnimNotify_Scripted; /* AnimNotify that calls PlayVoice function if Owner is Hat_Pawn. */ enum EPlayerVoice { PlayerVoice_Jump<DisplayName=Jump>, PlayerVoice_WallImpact<DisplayName=Wall impact>, PlayerVoice_Attack<DisplayName=Attack>, PlayerVoice_Hurt<DisplayName=Hurt>, PlayerVoice_HookshotCling<DisplayName=Hookshot cling>, PlayerVoice_MonitorClick<DisplayName=Monitor click>, PlayerVoice_Counting<DisplayName=Counting (0-9)>, PlayerVoice_CollectedAll<DisplayName=Collected all>, PlayerVoice_FreeCitizen<DisplayName=Freed citizen>, PlayerVoice_LongFallNoDmg<DisplayName=No damage long fall>, PlayerVoice_HelpAbilityHmm<DisplayName=Helper Hat hmm>, PlayerVoice_HatStitch<DisplayName=Stitching Hat (0-1)>, PlayerVoice_MurderClueGotcha<DisplayName=Gotcha Murder Clue>, PlayerVoice_HurtPant<DisplayName=Hurt pant>, PlayerVoice_NPCTease<DisplayName=NPC tease>, PlayerVoice_VacuumSpin<DisplayName=Rumbi spin>, PlayerVoice_ShopItemExamine<DisplayName=Examining shop item>, PlayerVoice_ShopLeave<DisplayName=Leaving shop>, PlayerVoice_ChemicalExplosion<DisplayName=Brewing Hat explosion>, PlayerVoice_HatStolen<DisplayName=Hat stolen>, PlayerVoice_DivaGreet<DisplayName=Diva greet>, PlayerVoice_TakePainting<DisplayName=Taking painting>, PlayerVoice_BurnPainting<DisplayName=Burning painting> }; var() SoundCue Voice; var(Player) bool UsePlayerScriptVoice<DisplayName=Use Player script voice (if owned by Player)>; var(Player) EPlayerVoice PlayerVoice<DisplayName=Player voice | EditCondition=UsePlayerScriptVoice>; var(Player) int PlayerVoiceArrayPosition<DisplayName=Voice list position | EditCondition=UsePlayerScriptVoice | UIMin=0>; var() float RelaxTime<DisplayName=Relax time | UIMin=0.0 | ClampMin=0.0>; var() bool IgnoreRelax<DisplayName=Ignore relax>; var() bool IsConversation<DisplayName=Is conversation>; event Notify(Actor Owner, AnimNodeSequence AnimSeqInstigator) { local SoundCue c; local Hat_Pawn p; local Hat_Player ply; p = Hat_Pawn(Owner); if (p == None) return; if (!UsePlayerScriptVoice || Hat_Player(p) == None) c = Voice; else { ply = Hat_Player(p); switch(PlayerVoice) { case PlayerVoice_Jump: c = ply.VoiceJump; break; case PlayerVoice_WallImpact: c = ply.VoiceWallImpact; break; case PlayerVoice_Attack: c = ply.VoiceAttack; break; case PlayerVoice_Hurt: c = ply.VoiceHurt; break; case PlayerVoice_HookshotCling: c = ply.VoiceHookshotCling; break; case PlayerVoice_MonitorClick: c = ply.VoiceMonitorClick; break; case PlayerVoice_Counting: c = ply.VoiceCounting[Min(Max(PlayerVoiceArrayPosition, 0), ArrayCount(ply.VoiceCounting)-1)]; break; case PlayerVoice_CollectedAll: c = ply.VoiceCollectedAll; break; case PlayerVoice_LongFallNoDmg: c = ply.VoiceLongFallNoDmg; break; case PlayerVoice_HelpAbilityHmm: c = ply.HelpAbilityHmm; break; case PlayerVoice_HatStitch: c = ply.VoiceHatStitch[Min(Max(PlayerVoiceArrayPosition, 0), ArrayCount(ply.VoiceHatStitch)-1)]; break; case PlayerVoice_MurderClueGotcha: c = ply.VoiceMurderClueGotcha; break; case PlayerVoice_HurtPant: c = ply.VoiceHurtPant; break; case PlayerVoice_NPCTease: c = ply.VoiceNPCTease; break; case PlayerVoice_VacuumSpin: c = ply.VoiceVacuumSpin; break; case PlayerVoice_ShopItemExamine: c = ply.VoiceShopItemExamine; break; case PlayerVoice_ShopLeave: c = ply.VoiceShopLeave; break; case PlayerVoice_ShopLeave: c = ply.VoiceShopLeave; break; case PlayerVoice_ChemicalExplosion: c = ply.VoiceChemicalExplosion; break; case PlayerVoice_HatStolen: c = ply.VoiceHatStolen; break; case PlayerVoice_DivaGreet: c = ply.VoiceDivaGreet; break; case PlayerVoice_TakePainting: c = ply.VoiceTakePainting; break; case PlayerVoice_BurnPainting: c = ply.VoiceBurnPainting; break; default: break; } } if (c != None) p.PlayVoice(c, RelaxTime, IgnoreRelax, IsConversation); }