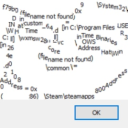
A Hat in Time Script Repository
(v0.5)
Code viewer
Deflecting
#Code
Short and simple snippet used for deflecting objects or players off a surface.
Short and simple snippet used for deflecting objects or players off a surface. Example: a ball hitting a wall, or a rolling player hitting a bumper.
Where it says KnockBackStregnth and DeflectForceLimit, those are variables you define in DefaultProperties.
DO NOT set DeflectForceLimit too high or the player or object WILL BE SENT OUT OF BOUNDS! Recommended values for DeflectForceLimit is 1500, and the recommended KnockBackStregnth is 2.
//in the bump function put this here simulated event Bump( Actor Other, PrimitiveComponent OtherComp, Vector HitNormal ) { if(Other.isA('Hat_Collectible_EnergyBit') || Other.isA('Hat_Collectible_HealthBit') || Other.isA('Hat_Collectible_PowerBit')) { return; //return as we don't want the rewarded pons to be yeeted } else { if (Other.Physics == Phys_Walking || Other.Physics == Phys_None) { Other.SetPhysics(Phys_Falling); } if (Other.Physics == Phys_Falling) { Other.Velocity = Deflector(Other.Velocity, HitNormal)*KnockBackStregnth; Other.Velocity = ClampLength(Other.Velocity, DeflectForceLimit); } } } //the function that does the real magic static function Vector Deflector(Vector vel, Vector HitNormal) { local Vector velN, velT; velN = HitNormal * (vel dot HitNormal); velT = vel - velN; return velT - velN; }