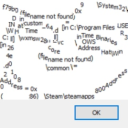
A Hat in Time Script Repository
(v0.5)
Code viewer
SS_SequenceAction_PlayerCounting
#Kismet
Plays counting sounds (including character specific), particle number and "Completed" output when reaching the limit, works with anything other than collectibles
To use this, you need to add an "Add Int" that tracks an int and ++ on it before counting sound plays, this int will play that specific sound and particles, make sure to add limit, the limit acts as the point where if it reaches that limit it plays the "Completed sound" and triggers the Completed output.
I've also added Stop which stops the sound playing, it checks if that sound based on the Counting number, so don't add int before casting stop on the node.
And volume just in case if you want to play with how high it plays.
// Made by SamiSha // Plays counting sounds from the Hat_Player file. Plays corresponding sounds for different playable characters too that have alternative SoundCues in those arrays. // CountingLevel is the number you want start with, by default its 1 up to 5, use "Add Int" to manipulate that int value so you can have it working consecutively with each trigger. // CollectedAllThreshold is the point where it instead plays the Completed sound and triggers the Completed output if you wanna do something extra with it. // Stop input stop any currently playing AudioComponent causes the Stopped Output to play. class SS_SequenceAction_PlayerCounting extends SequenceAction; var() int CountingLevel <Tooltip=The counting level plays the number which Hat Kid plays when collecting mission focused collectibles, e.g One, Two, Three, Four and Five>; var() int CollectedAllThreshold <Tooltip=The sound that plays when Hat Kid grabs all of them and shouts that she collected the necessary amount to finish that mini mission. This triggers the Completed output!>; var() float VolumeAmount <Tooltip=1 = 100%, 1.01 = 101%, 2 = 200% etc>; var() bool AllowParticles <Tooltip=Plays a Particle indicate the current number reached for visual clarity>; var Object Player; var AudioComponent CountingSound; var(Particles) ParticleSystem CollectNumberParticle; event Activated() { local Hat_Player ply; local Hat_PlayerController pc; local ParticleSystemComponent psc; pc = Hat_PlayerController(Player); ply = Hat_Player(pc.Pawn); if(InputLinks[0].bHasImpulse) { if (CountingLevel < CollectedAllThreshold) { if(CountingLevel < 6 && CountingLevel != 0) CountingSound = ply.CreateAudioComponent(ply.VoiceCounting[CountingLevel], true, true,,,true); if(ply.IsA('Hat_Player_CoPartner')) CountingSound.PitchMultiplier = 0.93f; CountingSound.VolumeMultiplier = VolumeAmount; if (CollectNumberParticle != None && CountingLevel < 10 && AllowParticles) { psc = ply.WorldInfo.MyEmitterPool.SpawnEmitter(CollectNumberParticle, ply.Location); psc.SetFloatParameter('Number', (CountingLevel-0.5f)/9.f); } OutputLinks[0].bHasImpulse = true; } else { CountingSound = ply.CreateAudioComponent(ply.VoiceCollectedAll, true, true,,,true); if(ply.IsA('Hat_Player_CoPartner')) CountingSound.PitchMultiplier = 0.93f; CountingSound.VolumeMultiplier = VolumeAmount; OutputLinks[1].bHasImpulse = true; } } if(InputLinks[1].bHasImpulse) { if(CountingSound != None) CountingSound.Stop(); OutputLinks[2].bHasImpulse = true; } } /* if (CollectNumberParticle != None && CollectedCount < 10) { psc = ply.WorldInfo.MyEmitterPool.SpawnEmitter(CollectNumberParticle, c.Location); psc.SetFloatParameter('Number', (CollectedCount-0.5f)/9.f); } */ // SOME PARTICLE I FOUND defaultproperties { ObjName="Play Counting Sounds"; ObjCategory="Player"; InputLinks(0)=(LinkDesc="In"); InputLinks(1)=(LinkDesc="Stop"); OutputLinks(0)=(LinkDesc="Out"); OutputLinks(1)=(LinkDesc="Completed"); OutputLinks(2)=(LinkDesc="Stopped"); VariableLinks(0)=(ExpectedType=class'SeqVar_Int',LinkDesc="Count",PropertyName=CountingLevel); VariableLinks(1)=(ExpectedType=class'SeqVar_Int',LinkDesc="Limit",PropertyName=CollectedAllThreshold); VariableLinks(2)=(ExpectedType=class'SeqVar_Float',LinkDesc="Volume",PropertyName=VolumeAmount); VariableLinks(3)=(ExpectedType=class'SeqVar_Object',LinkDesc="Target",PropertyName=Player); CollectNumberParticle = ParticleSystem'HatInTime_Items.ParticleSystems.VaultCodeCollected_Number' bAutoActivateOutputLinks = false; VolumeAmount = 1f; }