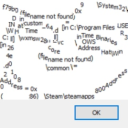
(v0.5)
Searching Objects on the Map Via Scripting
A small guide of different ways to do so.
It all revolves around AllActors/DynamicActors function calls which are available if the class this script is extending from is the Actor.uc
file, this code snippets will provide multiple code examples that should get the point across.
If the class this is being called from is not extending to Actor.uc
, you can always use the following cast to get a grab of those functions through the WorldInfo
class'Worldinfo'.static.GetWorldInfo()
// returns WorldInfo (which is extended to Actor.uc
so you can get those foreach calls).
The hard search object which takes an ID is not recommended for custom made maps as kismet does that already while having more self control yourself, hard search objects via ID is more relevant to snatching specific objects that are found in the official maps for specific map modifications or overhauling certain functionality and so on.
// Template function OBJECT_NAME GetOBJECT_NAME(int id) { local OBJECT_NAME obj; foreach class'Worldinfo'.static.GetWorldInfo().AllActors(class'OBJECT_NAME', obj) { if(!obj.IsA('OBJECT_NAME')) continue; if(string(obj.Name) ~= ("OBJECT_NAME_" $ id)) return obj; } return None; } // the this function can be called like this: GetOBJECT_NAME(5); // Returns an insance, so you better save it into a variable OR cast upon it if its only for one time objectNumber5 = GetOBJECT_NAME(5); // saved to a global/local variable GetOBJECT_NAME(5).ShutDown(); // one time cast, afterwards this instance is lost due not being saved objectNumber5.ShutDown(); // And yes, you can also do those function calls or changes on global/local variables. // Example from the above, this one looks up a specific volume type in the world, keep in mind ID for those volumes are never changed (unless I believe the map was streamed, though this is never been tested) function TriggerVolume GetTriggerVolume(int id) { local TriggerVolume tv; foreach class'Worldinfo'.static.GetWorldInfo().AllActors(class'TriggerVolume', tv) { if(!tv.IsA('TriggerVolume')) continue; if(string(tv.Name) ~= ("TriggerVolume_" $ id)) return tv; } return None; } // Returns the searched trigger volume in the map GetTriggerVolume(5); // Get all objects template function Array<OBJECT_NAME> GetOBJECT_NAMEs() { local Array<OBJECT_NAME> objects; local OBJECT_NAME obj; foreach class'Worldinfo'.static.GetWorldInfo().AllActors(class'OBJECT_NAME', obj) { if(!obj.IsA('OBJECT_NAME')) continue; objects.AddItem(obj); } return objects; // If it didn't find anything, this will return none... nonetheless :) } // Example using trigger volumes function Array<TriggerVolume> GetTriggerVolumes() { local Array<TriggerVolume> triggerVolumes; local TriggerVolume tv; foreach class'Worldinfo'.static.GetWorldInfo().AllActors(class'TriggerVolume', tv) { if(!tv.IsA('TriggerVolume')) continue; triggerVolumes.AddItem(tv); } return triggerVolumes; // If it didn't find anything, this will return none... nonetheless :) }