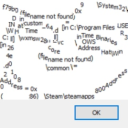
A Hat in Time Script Repository
(v0.5)
Code viewer
Inspect Items
#HUD
Inspect Items, with text implementation for custom text!
Similar to the Burger, Space Cows, Trains, and even drawing pencils, this behaves similarly to them, but allows you to input in texts through a kismet node, simply call the kismet, right clicking causes you to exit the HUD normally.
class SS_SequenceAction_InspectItem extends SequenceAction; var() String Header<autocomment=true>; var() Array<String> Descriptions; var Object Player; event Activated() { local Hat_Player plyr; local SS_HUDMenu_DecoratedInfo hud; plyr = GetPlayer(Player); if(plyr == None) return; if(InputLinks[0].bHasImpulse) { hud = SS_HUDMenu_DecoratedInfo(Hat_HUD(Hat_PlayerController(plyr.Controller).myHUD).OpenHUD(class'SS_HUDMenu_DecoratedInfo')); hud.SetDecoratedText(Hat_HUD(PlayerController(plyr.Controller).MyHUD), Header, Descriptions); hud.KismetInstigator = self; OutputLinks[0].bHasImpulse = true; } } function Hat_Player GetPlayer(Object p) { local Hat_Player ply; ply = Hat_Player(p); return ((ply != None && ply.IsA('Hat_Player')) ? ply : Hat_Player(Hat_PlayerController(p).Pawn)); } defaultproperties { ObjName="Inspect Item"; ObjCategory="Player"; InputLinks(0)=(LinkDesc="Inspect"); InputLinks(1)=(LinkDesc="Leave"); OutputLinks(0)=(LinkDesc="Out"); OutputLinks(1)=(LinkDesc="On Leaving"); VariableLinks(0)=(ExpectedType=class'SeqVar_Object',LinkDesc="Target",PropertyName=Player); bAutoActivateOutputLinks = false; }
/** * * Copyright 2012-2015 Gears for Breakfast ApS. All Rights Reserved. */ class SS_HUDMenu_DecoratedInfo extends Hat_HUDMenuEquipOtherItems; var Texture2D PresentTexture; var Texture2D SquareTexture; var float DescriptionFadeIn; var string LocalizedHeader; var Array<string> LocalizedDescription; var Array< class < Hat_Collectible_Important > > ScrambledAvailableItems; var transient bool ReentrantGuard; var SS_SequenceAction_InspectItem KismetInstigator; function OnOpenHUD(HUD H, optional string command) { // local array<string> desc; Super.OnOpenHUD(H, command); Hat_Player(H.PlayerOwner.Pawn).Taunt("Thinking"); Hat_PlayerController(H.PlayerOwner).CoopCriticalCountStack += 1; // desc.AddItem("sus"); //SetDecoratedText(H, "", desc); } function OnCloseHUD(HUD H) { Super.OnCloseHUD(H); Hat_Player(H.PlayerOwner.Pawn).Taunt(""); KismetInstigator.ForceActivateOutput(1); Hat_PlayerController(H.PlayerOwner).CoopCriticalCountStack -= 1; } function SetDecoratedText(HUD H, string header, Array<string> descriptions) { local int i; CurrentIndex = AvailableItems.Length > 0 ? FFloor((float(AvailableItems.Length)-0.5) / 2.0) : 0; SmoothIndex.X = CurrentIndex; SmoothIndex.Y = CurrentIndex; SmoothIndex.Z = 1; DescriptionFadeIn = 0; LocalizedHeader = header; LocalizedDescription.Length = 0; for (i = 0; i < descriptions.Length; i++) { LocalizedDescription.AddItem(Caps(descriptions[i])); } } function bool Tick(HUD H, float d) { if (!Super.Tick(H, d)) return false; if (DescriptionFadeIn >= 0) DescriptionFadeIn += d; return true; } function bool IsDoingCinematic() { return IsCompleted(); } // Required for the super class override!!! function bool RenderItemRows() { return false; } function bool IsCompleted() { return true; } function bool Render(HUD H) { if (!Super.Render(H)) return false; if (DescriptionFadeIn >= 0) RenderDescription(H, DescriptionFadeIn); return true; } function OnRenderIcon(HUD H, int index, float posx, float posy, float bopY, float IconScale, float SelectScale, Surface InTexture) { local float PresentSize; PresentSize = Lerp(SelectScale, 1.0, 0.6)*iconscale*0.8; DrawCenter(H, posx - iconscale*0.15, posy +iconscale*0.2 + (SelectScale-0.3)*iconscale*0.3, PresentSize, PresentSize, PresentTexture); Super.OnRenderIcon(H, index, posx, posy, BopY, iconScale, SelectScale, InTexture); } function bool DisablesCameraMovement(HUD H) { return false; } function bool OnClick(HUD H, bool release) { if (!release) { if (!IsCompleted() && CurrentIndex >= 0 && CurrentIndex < AvailableItems.Length && AvailableItems[CurrentIndex] != None) { return true; } else { CloseHUD(H, Class); return true; } } return Super.OnClick(H, release); } function bool OnAltClick(HUD H, bool release) { if(!release && !IsCompleted()) { CloseHUD(H, Class); return true; } else if (!release && IsCompleted()) { DescriptionFadeIn = DescriptionFadeIn >= 0 ? -1 : 0; } return Super.OnAltClick(H, release); } function RenderBounceLetter(HUD H, string s, float x, float y, float size, float angle, bool fadein) { local float pxwidth, pxheight; local Rotator r; H.Canvas.StrLen(s, pxwidth, pxheight); pxwidth *= size; pxheight *= size; if (fadein) { y -= size*angle*50; DrawTopLeftText(H.Canvas, s, x, y, size, size); } else { H.Canvas.PushTranslationMatrix(vect(1,0,0)*(x+pxwidth/2) + vect(0,1,0)*(y + pxheight)); r.Yaw = angle*65536; H.Canvas.PushRotationMatrix(r); DrawTopLeftText(H.Canvas, s, -pxwidth/2, -pxheight, size, size); H.Canvas.PopTransform(); H.Canvas.PopTransform(); } } function RenderBouncyText(HUD H, string s, float x, float y, float size, float letters, int lookahead, optional bool fadein) { local int staticletters, i; local float dynamicletter, alpha, invalpha, pxwidth, pxheight; staticletters = int(letters); dynamicletter = letters-staticletters; H.Canvas.SetDrawColor(255,255,255,200); if (staticletters > 0) DrawTopLeftText(H.Canvas, Left(s, staticletters), x, y, size, size); H.Canvas.StrLen(Left(s, staticletters), pxwidth, pxheight); x += pxwidth*size; // lookahead seems broken for desc if (fadein) return; for (i = 0; i < lookahead; i++) { alpha = dynamicletter/float(lookahead); alpha += (lookahead-i-1)*(1.0/float(lookahead)); if (alpha <= 0) break; if (staticletters+i >= Len(s)) break; invalpha = 1-alpha; H.Canvas.SetDrawColor(255,255,255,200 * (1-(invalpha**3))); RenderBounceLetter(H, Mid(s, staticletters+i,1), x, y, size, (invalpha**2)*0.15, fadein); H.Canvas.StrLen(Mid(s, staticletters+i,1), pxwidth, pxheight); x += pxwidth*size; } } function RenderDescription(HUD H, float in) { local float scale, headerletters, descletters; local string header; local int i, l; //local Array<string> desc; // black bottom bar H.Canvas.SetDrawColor(255,255,255,255); H.Canvas.SetPos(0, H.Canvas.ClipY - 270*0.5); H.Canvas.DrawRect(H.Canvas.ClipX, 270*0.5, HorizontalLineTexture); scale = FMin(H.Canvas.ClipX, H.Canvas.ClipY) * 0.0017; H.Canvas.SetDrawColor(255,255,255,225); header = LocalizedHeader; headerletters = in*35 - 5; H.Canvas.Font = class'Hat_FontInfo'.static.GetDefaultFont(header); RenderBouncyText(H, header, H.Canvas.ClipX*0.02, H.Canvas.ClipY*0.02, scale, headerletters, 5); scale = scale*0.35; l = 0; for (i = 0; i < LocalizedDescription.Length; i++) { descletters = (in-0.5)*200 - 20 - l; H.Canvas.Font = class'Hat_FontInfo'.static.GetDefaultFont(LocalizedDescription[i]); RenderBouncyText(H, LocalizedDescription[i], H.Canvas.ClipX*0.02, H.Canvas.ClipY*0.85 + scale*i*50, scale, descletters, 20, true); l += Len(LocalizedDescription[i]); } } defaultproperties { SquareTexture = Texture2D'HatInTime_Hud_ActSelect.Textures.decoration_square' DimSelected = true; PresentTexture = Texture2D'HatInTime_Hud_ItemIcons.Decorations.decoration_present' }