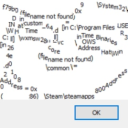
(v0.5)
Key Press Listeners Guide
A detailed how to, where you can add an event listener when pressing keys through a script.
There's a lot of to document, but this is done to add more features, this is mainly and widely used to add a new ability that overrides the Camera Snap button that is being utilized in A LOT OF MODS!!
When a button is pressed, they are passed through the function, which you can then trigger custom code, or override the input with a new input, causing for a lot of custom functionality to be utilized!
Add the following code into your Script, remember to read the important that can be found in the functions just so the script become compatible from where you are running this!
For obvious reasons, do not abuse the usage of event listener as they have the risk of being weaponized in making the game unplayable (since you can override all the presses), with that said, you've been warned!!!
// Add this at the top of the script, this is the core handler of the key event listener. var Interaction KeyCaptureInteraction; // Call this function at the beginning of the script to register the key event listener RegisterKeyEvent(); // Call this function when don't want to listen to key events, this is mostly done when you are about to destroy the script, recommended cleanup if you don't your console ot be massed with warnings. UnRegisterKeyEvent(); function RegisterKeyEvent() { local int iInput; local Hat_PlayerController pc; // IMPORTANT: // What is being passed in Hat_PlayerController() may vary depending from where this is setting up. // From a HUD: Add "HUD H" in the parameter above in the function, becoming RegisterKeyEvent(HUD H), then inside the Hat_PlayerController, pass "H.PlayerOwner". Keep in mind you need to pass the HUD into the function call!!! // From an Actor: Use GetALocalPlayerController(), this doesn't support Coop situations. // From a Pawn: All Pawns have a controller, simply pass "Controller" // From a Controller: Literally pass itself by writing "self" lol. // Note: the one being used here is done for a badge script. Hence "Instigator". pc = Hat_PlayerController(Instigator.Controller); KeyCaptureInteraction = new(pc) class'Interaction'; KeyCaptureInteraction.OnReceivedNativeInputKey = ReceivedNativeInputKey; // Add Axis inputs, this is done for joysticks or movement controls KeyCaptureInteraction.OnReceivedNativeInputAxis = ReceivedNativeInputAxis; iInput = pc.Interactions.Find(pc.PlayerInput); pc.Interactions.InsertItem(Max(iInput, 0), KeyCaptureInteraction); } function UnregisterKeyEvent() { local Hat_PlayerController pc; // IMPORTANT: // Read the important note in RegisterKeyEvent() function, same thing applies here. pc = Hat_PlayerController(Instigator.Controller); pc.Interactions.RemoveItem(KeyCaptureInteraction); KeyCaptureInteraction = None; pc = None; } /** * Provides script-only child classes the opportunity to handle input key events received from the viewport. * This delegate is ONLY called when input is being routed natively from the GameViewportClient * (i.e. NOT when unrealscript calls the InputKey native unrealscript function on this Interaction). * * @param ControllerId the controller that generated this input key event * @param Key the name of the key which an event occured for (KEY_Up, KEY_Down, etc.) * @param EventType the type of event which occured (pressed, released, etc.) * @param AmountDepressed for analog keys, the depression percent. * @param bGamepad input came from gamepad (ie xbox controller) * * @return return TRUE to indicate that the input event was handled. if the return value is TRUE, the input event will not * be processed by this Interaction's native code. */ /* SAMISHA ADD: * EventType reference sheet: * IE_Pressed - When pressing the button * IE_Released - When releasing the button * IE_Repeat - When holding the button * IE_DoubleClick - When double clicking in a succession * * For more info about Key names, check the IsPlayerKey function for a reference sheet! */ function bool ReceivedNativeInputKey(int ControllerId, name Key, EInputEvent EventType, float AmountDepressed, bool bGamepad) { // Hat_Menu_Start = Pause/Menu, don't override if you // IsPaused() a funciton found in the Controller class // IMPORTANT: // Read the comment in RegisterKeyEvent(), same thing applies. if(Hat_PlayerController(Instigator.Controller).IsPaused() || Key == 'Hat_Menu_Start') return false; // For more info about Key names, check the IsPlayerKey function for a reference sheet! if(Key == 'Hat_Player_CameraSnap' && EventType == IE_Pressed) { // Do stuff! } return false; } /** * Provides script-only child classes the opportunity to handle input axis events received from the viewport. * This delegate is ONLY called when input is being routed natively from the GameViewportClient * (i.e. NOT when unrealscript calls the InputKey native unrealscript function on this Interaction). * * @param ControllerId the controller that generated this input axis event * @param Key the name of the axis that moved, SAMISHA EDIT: Use Hat_Player_MoveX/Hat_Player_MoveY for universal support for controllers (keyboard and gamepad joysticks) * @param Delta the movement delta for the axis * @param DeltaTime the time (in seconds) since the last axis update. * @param bGamepad input came from gamepad (ie xbox controller) * * @return return TRUE to indicate that the input event was handled. if the return value is TRUE, the input event will not * be processed by this Interaction's native code. */ function bool ReceivedNativeInputAxis(int ControllerId, name Key, float Delta, float DeltaTime, optional bool bGamepad) { Switch(Key) { Case 'Hat_Player_MoveX': // Do stuff // This is for left/right movement of the joystick, A/D buttons on keyboard. break; Case 'Hat_Player_MoveY': // Do stuff // This is for down/up movement of the joystick, S/W buttons on keyboard. break; default: break; } return false; } // Custom function I made, check if its a player key, you can call this for um, things... to do with any button that is related to the game controls, or use this as a reference sheet for the universal controls. // Reminder, DO NOT PASS explicit key names e,g 'SpaceBar' as you will be supporting one controller instead of two (in this case example, keyboard jump button instead of the gamepad's) function bool IsPlayerKey(name Key) { switch(Key) { case 'Hat_Player_Attack': case 'Hat_Ability_Swap': case 'Hat_Player_CameraSnap': case 'Hat_Player_Jump': case 'Hat_Player_Crouch': case 'Hat_Player_Ability': case 'Hat_Player_Interact': case 'Hat_Hotkey_Up': // Taunt case 'Hat_Hotkey_Down': // Kiss case 'Hat_Hotkey_Left': case 'Hat_Hotkey_Right': case 'Hat_Player_Share': case 'Hat_Player_AbilitySwap': return true; // The pause key, I would highly recommend to never override this, else you are a meany!!! case 'Hat_Menu_Start': return false; default: return false; } }