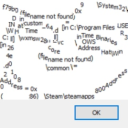
A Hat in Time Script Repository
(v0.5)
Code viewer
Detecting other mods that Asset-Replace Objects your mod already does
#Code
A function that sends info about other mods that override Asset-Replaces from your mod.
CheckOtherARMods function from this script sends info about every mod that Asset-Replaces same objects as your mod does. The info is sent both into Launch_2.log file and into console (using GameInfo's Broadcast). Can be used to warn players or other modders in case something important is being Asset-Replaced. Both functions should be placed in your GameMod-extending Class.
static function CheckOtherARMods() { local Array<GameModInfo> ModList; local Array<string> Messages; local string s; local GameModInfo ThisModInfo; local int i, j, k; if (!GetClassMod(default.Class, ThisModInfo)) return; if (ThisModInfo.AssetReplaces.Length < 1) return; ModList = GetModList(); for (i = 0; i < ModList.Length; i++) { if (ModList[i].ModClass == None) continue; if (ModList[i].ModClass == default.Class) continue; if (!ModList[i].IsSubscribed) continue; if (ModList[i].IsDownloading) continue; if (!ModList[i].IsEnabled) continue; for (j = 0; j < ModList[i].AssetReplaces.Length; j++) { for (k = 0; k < ThisModInfo.AssetReplaces.Length; k++) { if (ModList[i].AssetReplaces[j].TargetAsset ~= ThisModInfo.AssetReplaces[k].TargetAsset) Messages.AddItem("Detected another mod that replaces"@ThisModInfo.AssetReplaces[k].TargetAsset$":"@ModList[i].Name@"(script:"@PathName(ModList[i].ModClass)$"). Replaced Objects can have unexpected behaviour!"); } } } for (i = 0; i < Messages.Length; i++) { if (ThisModInfo.Name != "") s $= "["$ThisModInfo.Name$"] Warning: "; else s $= "["$default.Class$"] Warning: "; if (Messages[i] == "") s $= "???"; else s $= Messages[i]; if (i != Messages.Length-1) s $= "\n"; } if (s != "") SendMessage(s); } static function SendMessage(string Message) { local WorldInfo wi; if (Message == "") return; LogMod(Message); wi = class'WorldInfo'.static.GetWorldInfo(); if (wi != None && wi.Game != None) wi.Game.Broadcast(wi, Message); }