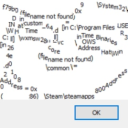
A Hat in Time Script Repository
(v0.5)
Code viewer
Shara_SteamID_Tools
Modified @ 2024-07-24 22:12:42
A set of functions for dealing with players' Steam IDs, indexes and Controllers.
Have you ever imagined player riding on Vehicle? But did you know that when it's on Vehicle, it does not have Controller assigned to it? Controller is assigned to Vehicle, not player. Now imagine how many other what ifs this monstrosity of a game has? I am trying to overcome them by making my own monstrosity of a script that has a lot of functions to deal with player's Steam IDs, indexes, and Controllers.
class Shara_SteamID_Tools extends Object abstract; /* This class includes functions that are dealing with players' Steam IDs, indexes and Controllers. This is a base file. Please add suffix at the end of class name to differentiate classes and remove this line and maybe add your own (describing what mod it's used in). All functions are written by Shararamosh. Last edited: 31.01.2024 0:59 GMT+3. */ final static function string GetMySteamID() //Returns your Steam ID. { local OnlineSubsystemCommonImpl OnlineSubsystem; OnlineSubsystem = OnlineSubsystemCommonImpl(class'GameEngine'.static.GetOnlineSubsystem()); if (OnlineSubsystem == None) return ""; return OnlineSubsystem.GetUserCommunityID(); } final static function string GetOtherSteamID(Hat_GhostPartyPlayerStateBase s) //Returns Steam ID from Hat_GhostPartyPlayerStateBase. { if (s != None) return s.GetNetworkingIDString(); return ""; } final static function bool GetPlayerInfo(Actor a, out string SteamID, out int PlayerIndex) //Returns Hat_Player's and Hat_GhostPartyPlayer's Steam ID and player index as out parameters and true if these parameters have passed proof-check. { local Hat_Player ply; local Hat_GhostPartyPlayer gpp; SteamID = ""; PlayerIndex = -1; gpp = Hat_GhostPartyPlayer(a); if (gpp != None) { if (gpp.PlayerState != None) { SteamID = GetOtherSteamID(gpp.PlayerState); PlayerIndex = gpp.PlayerState.SubID; return IsCorrectPlayerIndex(PlayerIndex) && IsCorrectSteamID(SteamID); } return false; } ply = GetPlayerPawn(a); if (ply == None) return false; PlayerIndex = GetPawnPlayerIndex(ply); SteamID = GetMySteamID(); return IsCorrectPlayerIndex(PlayerIndex) && IsCorrectSteamID(SteamID); } final static function Controller GetController(Object o) //Returns Controller. Input parameter can be HUD, Pawn, PlayerController and Player. { local HUD H; local Pawn p; local Controller c; local Player EnginePlayer; c = Controller(o); if (c != None) return c; p = Pawn(o); if (p != None) return GetPawnController(p); H = HUD(o); if (H != None) return H.PlayerOwner; EnginePlayer = Player(o); if (EnginePlayer != None) return EnginePlayer.Actor; return None; } final static function PlayerController GetPlayerController(Object o) //Returns PlayerController. Input parameter can be HUD, Pawn, PlayerController and Player. { local HUD H; local Pawn p; local PlayerController pc; local Player EnginePlayer; pc = PlayerController(o); if (pc != None) return pc; p = Pawn(o); if (p != None) return GetPawnPlayerController(p); H = HUD(o); if (H != None) return H.PlayerOwner; EnginePlayer = Player(o); if (EnginePlayer != None) return EnginePlayer.Actor; return None; } final static function Controller GetPawnController(Pawn p, optional out Array<Pawn> IteratedPawns) //Will return the first found Controller, not necessarily PlayerController. IteratedPawns is used to prevent infinite loop when iterating Vehicle's Driver and Pawn's DrivenVehicle. { local Vehicle v; local Controller c; if (p == None) return None; if (p.Controller != None) return p.Controller; if (IteratedPawns.Find(p) < 0) IteratedPawns.AddItem(p); else return None; v = Vehicle(p); if (v != None) { c = GetPawnController(v.Driver, IteratedPawns); if (c != None) return c; } return GetPawnController(p.DrivenVehicle, IteratedPawns); } final static function PlayerController GetPawnPlayerController(Pawn p, optional out Array<Pawn> IteratedPawns) //Returns PlayerController for Pawn. Will check Pawn itself and DrivenVehicle for PlayerController. IteratedPawns is used to prevent infinite loop when iterating Vehicle's Driver and Pawn's DrivenVehicle. { local Vehicle v; local PlayerController pc; if (p == None) return None; pc = PlayerController(p.Controller); if (pc != None) return pc; if (IteratedPawns.Find(p) < 0) IteratedPawns.AddItem(p); else return None; v = Vehicle(p); if (v != None) { pc = GetPawnPlayerController(v.Driver, IteratedPawns); if (pc != None) return pc; } return GetPawnPlayerController(p.DrivenVehicle, IteratedPawns); } final static function int GetPawnPlayerIndex(Pawn p) //Returns player index of Pawn by first finding PlayerController of it. { return GetPlayerIndex(GetPawnPlayerController(p)); } final static function int GetPlayerIndex(PlayerController pc) //Returns player index of PlayerController by either casting to Hat_PlayerController_Base or GamePlayerController or by checking Engine.GamePlayers Array. { local int i; local Engine e; local GamePlayerController gpc; local Hat_PlayerController_Base hpcb; if (pc == None) return -1; gpc = GamePlayerController(pc); if (gpc != None) { hpcb = Hat_PlayerController_Base(gpc); if (hpcb != None) return hpcb.GetPlayerIndex(); return gpc.GetUIPlayerIndex(); } e = class'Engine'.static.GetEngine(); if (e == None) return -1; for (i = 0; i < e.GamePlayers.Length; i++) { if (e.GamePlayers[i] == None) continue; if (e.GamePlayers[i].Actor == pc) return i; } return -1; } final static function PlayerController GetPlayerByIndex(int PlayerIndex) //Returns PlayerController that corresponds to this player index. { local Engine e; local PlayerController pc; if (!IsCorrectPlayerIndex(PlayerIndex)) return None; pc = class'Hat_PlayerController_Base'.static.GetPlayerByIndex(PlayerIndex); if (pc != None) return pc; e = class'Engine'.static.GetEngine(); if (e == None) return None; if (e.GamePlayers.Length <= PlayerIndex) return None; if (e.GamePlayers[PlayerIndex] == None) return None; return e.GamePlayers[PlayerIndex].Actor; } final static function Pawn GetPawn(Object o) //Returns ANY Pawn, including Vehicle and Hat_Player. Input parameter can be Pawn, Controller, HUD, PlayerController and Player. { local HUD H; local Pawn p; local Controller c; local Player EnginePlayer; if (o == None) return None; p = Pawn(o); if (p != None) return p; c = Controller(o); if (c != None) return c.Pawn; H = HUD(o); if (H != None) return GetPawn(H.PlayerOwner); EnginePlayer = Player(o); if (EnginePlayer != None) return GetPawn(EnginePlayer.Actor); return None; } final static function Pawn GetNonVehiclePawn(Object o, optional out Array<Vehicle> IteratedVehicles) //Returns ONLY NON-VEHICLE Pawn, including Hat_Player. Uses GetPawn function to get the result and then checks whether it's a Vehicle. IteratedPawns is used to prevent infinite loop when iterating Vehicle's Driver and Pawn's DrivenVehicle. { local Pawn p; local Vehicle v; p = GetPawn(o); if (p == None) return None; v = Vehicle(p); if (v == None) return p; if (IteratedVehicles.Find(v) < 0) //Preventing a chain of Vehicles that have Driver set as another Vehicle (or itself). This should NEVER occur. IteratedVehicles.AddItem(v); else return None; return GetNonVehiclePawn(v.Driver, IteratedVehicles); } final static function Hat_Player GetPlayerPawn(Object o) //Returns Hat_Player Actor. Simply casts result of GetNonVehiclePawn to Hat_Player. { return Hat_Player(GetNonVehiclePawn(o)); } final static function int GetOtherPlayerIndex(Hat_Player ply) //Returns player index of another player (in Co-op or whatever). { local int i; local Engine e; local PlayerController pc; local Hat_PlayerController_Base hpcb; if (ply == None) return -1; pc = GetPawnPlayerController(ply); if (pc == None) return -1; hpcb = Hat_PlayerController_Base(pc); if (hpcb != None) return hpcb.GetOtherPlayerIndex(); e = class'Engine'.static.GetEngine(); if (e == None) return -1; for (i = 0; i < e.GamePlayers.Length; i++) { if (e.GamePlayers[i] == None) continue; if (e.GamePlayers[i].Actor == None) continue; if (e.GamePlayers[i].Actor == pc) continue; return i; } return -1; } final static function bool IsCorrectPlayerIndex(int n) //Very obvious check: player index can't be lower than 0. { if (n < 0) return false; return true; } final static function bool IsCorrectSteamID(string s) //Checks if the length is exactly 17 symbols and each symbol is a 0-9 number. { if (Len(s) != 17) return false; if (!IsStringNumber(s)) return false; return true; } final static function bool IsCharNumber(string s) //Is this exactly a 0-9 number? { switch(s) { case "0": case "1": case "2": case "3": case "4": case "5": case "6": case "7": case "8": case "9": return true; default: return false; } } final static function bool IsStringNumber(string s) //Is whole string a number? { local int i; local string StringLetter; for (i = 0; i < Len(s); i++) { StringLetter = Mid(s, i, 1); if (IsCharNumber(StringLetter)) continue; return false; } return true; } final static function bool WhichSteamIDIsBigger(string s1, string s2, out string LongerID) //GUYS, WE HAVE KILLED MODDING!!! REMEMBER??? That's why we can't have Steam IDs as integers! :hueh: { local int i; local string s1Letter, s2Letter; local Array<int> s1Chars, s2Chars; LongerID = ""; if (Len(s1) != 17 || Len(s2) != 17) return false; for (i = 0; i < 17; i++) { s1Letter = Mid(s1, i, 1); s2Letter = Mid(s2, i, 1); if (!IsCharNumber(s1Letter) || !IsCharNumber(s2Letter)) return false; s1Chars.AddItem(int(s1Letter)); s2Chars.AddItem(int(s2Letter)); } for (i = 0; i < 17; i++) { if (s1Chars[i] > s2Chars[i]) { LongerID = s1; return true; } if (s2Chars[i] > s1Chars[i]) { LongerID = s2; return true; } } return false; }