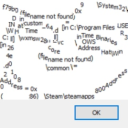
A Hat in Time Script Repository
(v0.5)
Code viewer
Custom Death
#Kismet
A simple system designed to implement custom death via Kismet.
To implement a quicky here's an example:
- Give the Status Effect "SS_StatusEffect_FakeBadge" to the player.
- Add a Remote Event called "OnCustomDeath", set the Max Trigger Count from 0 to 1.
- Upon taking fatal damage the Remote Event will be triggered
- Implement a custom death from the Remote Event output
Tip: Use the Console Command kismet to implement a way to reload the map by inputting "restartlevel" in the kismet node
Image demo:
class SS_Ability_Scriptable extends Hat_Ability_Automatic; const KISMET_CALL_NAME = 'OnCustomDeath'; simulated function PostBeginPlay() { Super.PostBeginPlay(); } function ItemRemovedFromInvManager() { Super.ItemRemovedFromInvManager(); } function OnTakeDamage(out int Damage, Controller InstigatedBy, vector HitLocation, out vector Momentum, class<DamageType> DamageType, optional TraceHitInfo HitInfo, optional Actor DamageCauser) { if(Instigator.Health - Damage <= 0) { Damage = 0; SetHealthTransition(GetHealthHUD(), PlayerController(Instigator.Controller), Instigator.Health, 0); CallRemoteEvent(KISMET_CALL_NAME, Instigator.Controller); } } simulated function Tick(float d) { // If you gonna ban the badge, comment this out. (saves like itsy bitsy performance that does absolutely NOTHING) Hat_InventoryManager(Hat_Player(Instigator).InvManager).Badges.Sort(SortPriority); } // Fixes one hit hero overriding revive delegate int SortPriority(Hat_Ability A, Hat_Ability B) { if (A.IsA(Class.Name)) return -1; return 0; } function SetHealthTransition(Hat_HUDElementHealth h, PlayerController c, int start, int finish, optional float multiplier = 1.0f) { local InterpCurveFloat Curve; h.HealthMaterialInstance.ClearParameterValues(); h.HealthMaterialInstance.SetScalarParameterValue('MaxHealth', h.GetPlayerMaxHealth(c.MyHUD)); Curve = class'Hat_Math'.static.GenerateCurveFloat(start, finish, 0.2f * multiplier); h.HealthMaterialInstance.SetScalarCurveParameterValue('CurrentHealth', Curve); h.HealthMaterialInstance.SetScalarStartTime('CurrentHealth', 0.0); } function Hat_HUDElementHealth GetHealthHUD() { local Hat_HUDElementHealth h; local Hat_Player plyr; local PlayerController c; plyr = Hat_Player(Owner); c = plyr.Controller != None ? PlayerController(plyr.Controller) : None; if (c != None && c.MyHUD != None && c.MyHUD.IsA('Hat_HUD')) h = Hat_HUDElementHealth(Hat_HUD(c.MyHUD).OpenHUD(class'Hat_HUDElementHealth', "Display")); return h != None ? h : None; } static function CallRemoteEvent(Name RemoteEventName, Actor InOriginator, optional Actor InInstigator) { local int i; local Sequence GameSeq; local array<SequenceObject> AllSeqEvents; if(String(RemoteEventName) ~= "") return; if(InInstigator == None) InInstigator = InOriginator; GameSeq = Class'WorldInfo'.static.GetWorldInfo().GetGameSequence(); if(GameSeq == None) return; GameSeq.FindSeqObjectsByClass(class'SeqEvent_RemoteEvent', true, AllSeqEvents); for(i=0; i < AllSeqEvents.Length; i++) { if(SeqEvent_RemoteEvent(AllSeqEvents[i]).EventName != RemoteEventName) continue; SequenceEvent(AllSeqEvents[i]).CheckActivate(InOriginator, InInstigator); } } defaultproperties { Begin Object Name=Mesh2 Materials(0) = None; End Object HUDIcon = None; CosmeticItemName = "SSScriptableLOLXD"; Description(0) = "SSScriptableLOLXDDesc0"; }
Class SS_StatusEffect_FakeBadge extends Hat_StatusEffect; var Inventory fakeBadge; function OnAdded(Actor a) { Super.OnAdded(a); fakeBadge = Owner.Spawn(Class'SS_Ability_Scriptable',,, vect(0,0,0)); Hat_InventoryManager(Hat_Player(Owner).InvManager).AddInventory(fakeBadge); } simulated function OnRemoved(Actor a) { Hat_InventoryManager(Hat_Player(Owner).InvManager).RemoveFromInventory(fakeBadge); fakeBadge.Destroy(); fakeBadge = None; Super.OnRemoved(a); } defaultproperties { Duration = -1; }