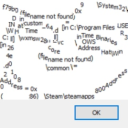
(v0.5)
Enable Post Process
A Kismet node for toggling post process effects, specifically MaterialEffects.
This a Kismet node that toggles the visibility of post process effects, specially MaterialEffects. It will find the post process effect based on the specified name and change its visibility. There is also support for animating and manipulating the effect's material parameters and even overriding the material itself.
Properties Overview
Effect Name: The name of the post process effect. Material Override: This is the material that will replace the effect's old material. Animate: Animate and manipulate the effect's material parameters using a curve. Animate Parameter: The material parameter to animate. This should ideally be a scalar parameter to ensure compatibility. Animate Curve: The interpolation curve used to animate the parameter. It is usually linear, but it can also be a proper exponential/easing curve if you're willing to mess around with the curve's points or copy one from a curve editor somewhere else in the editor. I think you could use a Matinee to animate a material parameter/a post process effect, but uh.... ignore that. Hide After Animating: Hide the effect once the animating finishes. You should only use this if your effect's material is very performance taxing and expensive to render. Don't use this if you have an Nvidia RTX 40000 or something because it won't make your game run faster.
/* Jlint_SeqAct_EnablePostProcess Toggle any post process effect/MaterialEffect that is in the current post processing chain - Toggle effects for a single player or everyone - Can input an effect name from a kismet variable or node properties - Makes effects gradually appear(just as long as they have the right material setup) - Overrides preexisting post process effects with your own materials Some code borrowed from Hat_SnatcherContract_DeathWish_RiftCollapse & Hat_HUD */ class Jlint_SeqAct_EnablePostProcess extends SequenceAction; var() string EffectName<ToolTip="The post process/material effect's name.">; var() Material MaterialOverride<ToolTip="If not empty, change the effect's material to this.">; var() bool Animate; //Animate the effect? var() string AnimateParameter<EditCondition=Animate|ToolTip="Effect material parameter to animate. Ideally, this should be a scalar parameter.">; var() InterpCurveFloat AnimateCurve<EditCondition=Animate|ToolTip="Interpolation curve to use when animating effects.">; var() bool HideAfterAnimating<EditCondition=Animate|ToolTip="Hide the effect after animating it? Use this for slightly more efficient rendering if you fade out an effect.">; static event int GetObjClassVersion() { return Super.GetObjClassVersion()+4; } defaultproperties { ObjName="Toggle Post Process Effect" ObjCategory="Visuals" InputLinks(0)=(LinkDesc="Show") InputLinks(1)=(LinkDesc="Hide") InputLinks(2)=(LinkDesc="Toggle") VariableLinks(1)=(ExpectedType=class'SeqVar_String',LinkDesc="Effect Name",PropertyName=EffectName) bCallHandler = false; AnimateParameter = "Alpha"; } function HidePostProcessEffect(Object Player) { local MaterialEffect Effect; if (Hat_PlayerController(Player) == None) return; Effect = MaterialEffect(LocalPlayer(Hat_PlayerController(Player).Player).PlayerPostProcess.FindPostProcessEffect(Name(EffectName))); Effect.bShowInGame = false; } event Activated() { local Object Player; local MaterialEffect Effect; local MaterialInstanceTimeVarying EffectMat; foreach Targets(Player) { if (Hat_PlayerController(Player) == None) continue; Effect = MaterialEffect(LocalPlayer(Hat_PlayerController(Player).Player).PlayerPostProcess.FindPostProcessEffect(Name(EffectName))); if (Effect == None) continue; if (MaterialOverride != None) Effect.SetMaterial(MaterialOverride); if (Animate) { EffectMat = new(Effect) class'MaterialInstanceTimeVarying'; EffectMat.SetParent(Effect.GetMaterial()); if (EffectMat != None) { Effect.SetMaterial(EffectMat); EffectMat.SetScalarCurveParameterValue(Name(AnimateParameter), AnimateCurve); EffectMat.SetScalarStartTime(Name(AnimateParameter), 0); } if (HideAfterAnimating) GetWorldInfo().Game.SetTimer(AnimateCurve.Points[AnimateCurve.Points.Length - 1].InVal, false, NameOf(HidePostProcessEffect), self, Player); } if (InputLinks[0].bHasImpulse) Effect.bShowInGame = true; else if (InputLinks[1].bHasImpulse) Effect.bShowInGame = false; else if (InputLinks[2].bHasImpulse) Effect.bShowInGame = !Effect.bShowInGame; } }