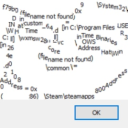
A Hat in Time Script Repository
(v0.5)
Code viewer
Extended Act/Level Bit nodes
#Kismet
A few extra nodes for reading/deleting Act Bits and Level Bits!
This adds 4 new nodes:
- Get Level Bit - Gets the value currently stored by a Level Bit (usually set with AbsoluteValue=True)
- Get Act Bit - Same as above, but for Act Bits
- Delete All Level Bits - Deletes all Level Bits from the specified maps (if unspecified, deletes from the current map)
- Delete All Act Bits - Same as above, but for Act Bits
NOTE: You may want to edit the value of ObjCategory to something unique, so you can tell these nodes apart from other copies in other mods.
/** * Kismet action responsible for deleting all current act bits. * Useful for simulating a level reset. */ class Drew_SeqAct_DeleteAllActBits extends SequenceAction; event Activated() { local Hat_SaveGame SG; SG = Hat_SaveGame(class'Hat_SaveBitHelper'.static.GetSaveGame()); if (SG != None) { SG.ActBits.Length = 0; } } defaultproperties { ObjName = "Delete All Act Bits" ObjCategory = "Give me a custom category!" bCallHandler = false VariableLinks.Empty }
/** * Kismet action responsible for deleting all (or all prefixed) level bits from one or more levels. * Useful for resetting the """save file""" of a mod. */ class Drew_SeqAct_DeleteAllLevelBits extends SequenceAction; /** Maps from which to delete level bits. If empty, the current map will be used. */ var() Array<string> Maps; /** If not empty, only level bits with this prefix will be deleted. */ var() string WithPrefix<autocomment=true>; event Activated() { local Hat_SaveGame_Base SG; local int i; if (Maps.Length == 0) { Maps.AddItem(class'Hat_SaveBitHelper'.static.GetCorrectedMapFilename()); } SG = class'Hat_SaveBitHelper'.static.GetSaveGame(); for (i = 0; i < Maps.Length; i++) { if (WithPrefix != "") class'Hat_SaveBitHelper'.static.ResetLevelBitsByPrefix(WithPrefix, Maps[i], SG); else class'Hat_SaveBitHelper'.static.ResetLevelBitsForLevel(Maps[i], SG); } } defaultproperties { ObjName = "Delete All Level Bits" ObjCategory = "Give me a custom category!" bCallHandler = false bSuppressAutoComment = false VariableLinks.Empty }
/** * Gets the integer that was saved in an act bit (likely with AbsoluteValue=True). */ class Drew_SeqAct_GetActBit extends SequenceAction; /** Id to get the act bit from. */ var() String Id<autocomment=true>; var int Value; event Activated() { if (Id == "") return; Value = class'Hat_SaveBitHelper'.static.GetActBits(Id); } defaultproperties { ObjName = "Get Act Bit" ObjCategory = "Give me a custom category!" bCallHandler = false bSuppressAutoComment = false VariableLinks.Empty VariableLinks(0) = (ExpectedType=class'SeqVar_Int', LinkDesc="Value", PropertyName=Value, bWriteable=true) }
/** * Gets the integer that was saved in a level bit (likely with AbsoluteValue=True). */ class Drew_SeqAct_GetLevelBit extends SequenceAction; /** Id to get the level bit from. */ var() String Id<autocomment=true>; /* Map to get the level bit from. Leave blank to get from the currently loaded map. */ var() String Map; var int Value; event Activated() { if (Id == "") return; Value = class'Hat_SaveBitHelper'.static.GetLevelBits(Id, Map); } defaultproperties { ObjName = "Get Level Bit" ObjCategory = "Give me a custom category!" bCallHandler = false bSuppressAutoComment = false VariableLinks.Empty VariableLinks(0) = (ExpectedType=class'SeqVar_Int', LinkDesc="Value", PropertyName=Value, bWriteable=true) }